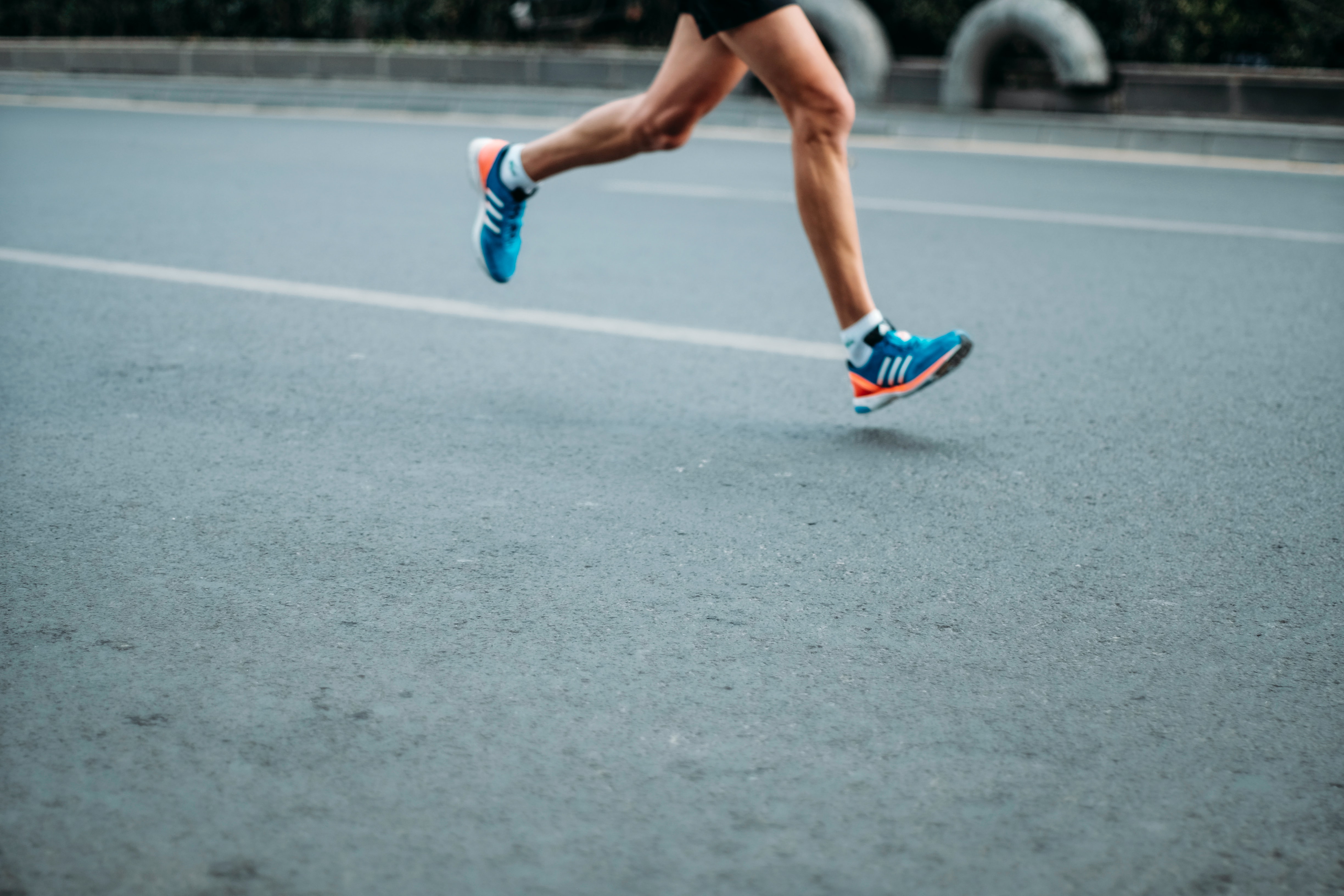
Running Django with nginx (on webfaction)
Case:
You’re using **virtualenv **and have used apache with a config somewhat like this.
Problem: Apache ‘goes to sleep’
Reason:
Apache caches your wsgi application, and if it’s not used within a certain amount of time, it has to reload. And this takes like.. 10-15 seconds.
Solution:
Use nginx instead:
Set up a custom application, and install nginx + uwsgi to point to your original application like this.
Potential new problem 2: Doesn’t find python – Can happen if you’ve installed the python version manually.
build_uwsgi.sh: line 41: python3.4: command not found
Solution 2: specify path to python instead of python version in build*uwsgi.sh *(you can find it with ‘which python3.4’ if you’re unsure)_
Potential new problem 3: Doesn’t find application. Can happen if you’re using virutalenv. Website responds with:
uWSGI Error
Python application not found
Solution 3: activate virtualenv in wsgi.py like this
import sys, os
virtualenv_root = os.path.expanduser('~/webapps/your_app/env')
activate_this = "%s/bin/activate_this.py" % virtualenv_root
# Python 2
# execfile(activate_this, dict(__file__=activate_this))
# Python 3
with open(activate_this) as f:
code = compile(f.read(), activate_this, 'exec')
exec(code, dict(__file__=activate_this))
workspace = os.path.expanduser('~/webapps/your_app/your_app')
sys.path.insert(0,workspace)
os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'your_app.settings')
# Older django
# import django.core.handlers.wsgi
# application = django.core.handlers.wsgi.WSGIHandler()
# Django 1.7+
from django.core.wsgi import get_wsgi_application
# Python 2
# application = get_wsgi_application()
# Python 3
from dj_static import Cling
application = Cling(get_wsgi_application())
Potential disappointment 4: nginx was not really faster – if this happens, you’ve probably not enabled threading support for uwsgi.
Solution 4: add the flag --enable-threading
.
For more flags, e.g. --workers
to set number of processes spawned, see uwsgi-docs.