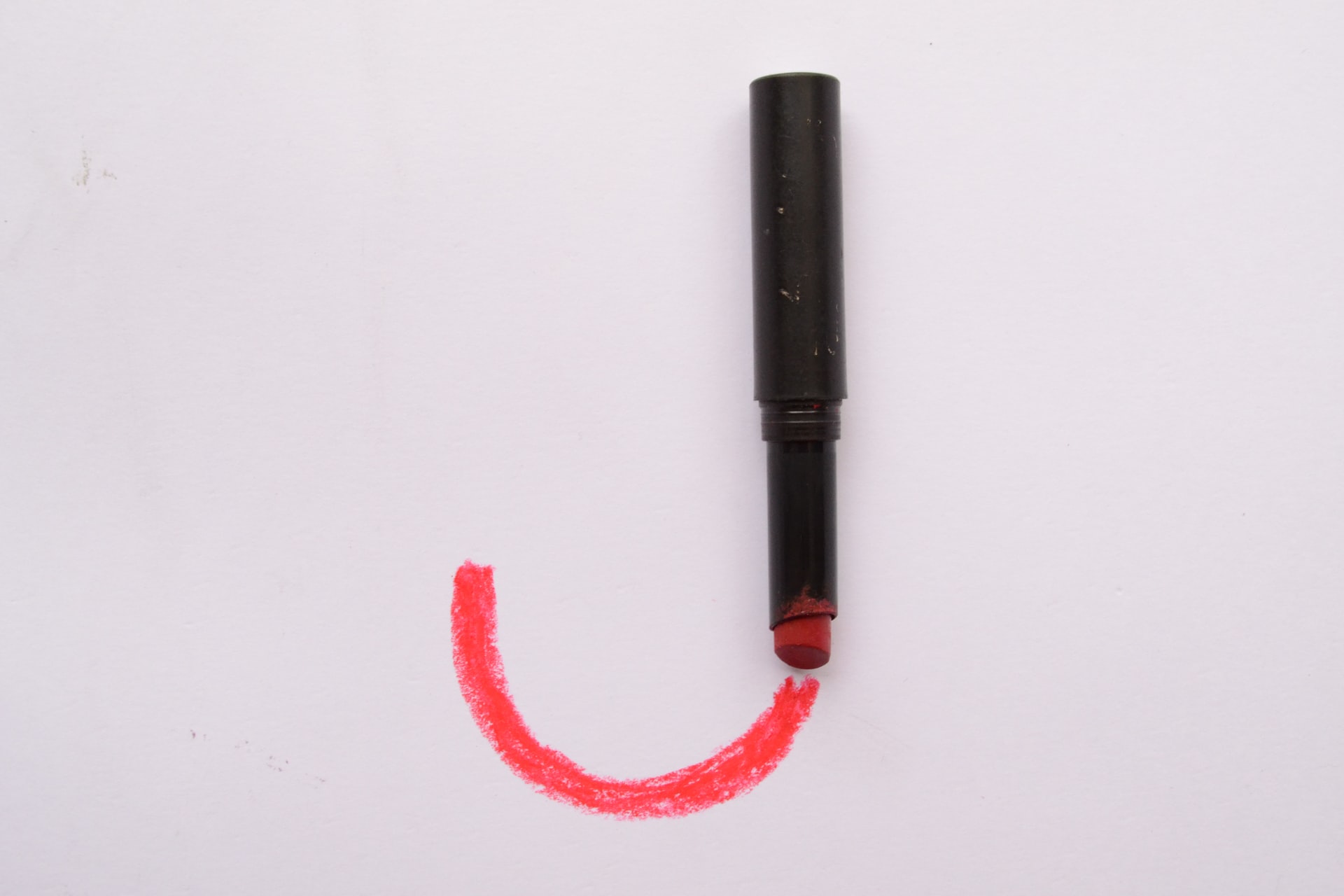
Migrating to React Redux hooks
Going from mapStateToProps
and mapStateToDispatch
to useDispatch, useSelector
or custom hooks: What’s the benefits? Does typing inference work?
This post is a step-by-step description of how I tested and came to the conclusions. The code below exists at github.com/tomfa/redux-hooks/, and I’ll be referencing commits as I go along.
Warning: Post is long. There is a TLDR at the bottom.
First: How is Redux doing?
Good, its NPM downloads has increased about 50% the last 12 months, same rate as React. So it seems safe to use: Reacts useContext and useReducer hooks, nor apollo-client
have made redux obsolete.
Plan
-
Set up a React Redux with Typescript
-
Implement some redux state, and implement UI using MapStateToProps and MapDispatchToProps. (MapXToProps from now on.)
-
Swap to using hooks.
Part I: Set up React Redux with Typescript
Install react with redux
npx create-react-app redux-hooks --template redux
And then run it:
yarn start
Sweet. The browser should show you something ala the above
Add typescript
Add types and the compiler (666f61)
yarn add -D \
typescript \
@types/node \
@types/react \
@types/react-dom \
@types/jest \
@types/react-redux
And rename all .js(x)
to .ts(x)
files (54bfd7). You could do this manually (there’s only ~10 files), or with the bash snippet here:
for x in $(find ./src -name \*.js); do
mv $x $(echo "$x" | sed 's/\.js$/.ts/')
done
for x in $(find ./src -name \*.tsx); do
mv $x $(echo "$x" | sed 's/\.tsx$/.jsx/')
done
Ok, sweet. Let’s add a tsconfig.json
with e.g. the following contents (8b76f82):
{
"compilerOptions": {
"target": "es5",
"lib": ["dom", "dom.iterable", "esnext"],
"allowJs": true,
"skipLibCheck": true,
"esModuleInterop": true,
"allowSyntheticDefaultImports": true,
"strict": true,
"forceConsistentCasingInFileNames": true,
"module": "esnext",
"moduleResolution": "node",
"resolveJsonModule": true,
"isolatedModules": true,
"noEmit": true,
"jsx": "react"
},
"include": ["src"]
}
This config above is from react-starter —template typescript:
General hygenic setup
Get the Redux browser extension
Get the browser extension (Chrome, Firefox) for Redux dev tools. It’s incredibly useful.
Click around and see how the log responds to your different clicks. Note how you can inspect the state, the action, the diff and export a test case (among other cool things).
Part II: Add some state
I decided to copy paste the state example from the redux.js recipe: Usage with TypeScript.
The app is a simple Chat app, where it consists of two main UI components:
ChatInput
ChatHistory
Some changes were made to the example code base, primarily to have self contained components without props that were using mapStateToProps and mapDispatchToProps. Below is the ChatInputComponent.tsx
.
The connected components can be viewed in the diff e877b50…6efc2a2.
import React from "react";
import { RootState } from "../../store";
import { Dispatch } from "redux";
import { connect } from "react-redux";
import { sendMessage, updateMessage } from "../../store/chat/actions";
import "./ChatInput.css";
interface OwnProps {}
type DispatchProps = ReturnType<typeof mapDispatchToProps>;
type StateProps = ReturnType<typeof mapStateToProps>;
type Props = DispatchProps & StateProps & OwnProps;
const ChatInterface: React.FC<Props> = ({ user, message, updateMessage, send }) => {
const onChange = (event: React.SyntheticEvent<{ value: string }>) => {
updateMessage(event.currentTarget.value);
};
function keyPress(e: React.KeyboardEvent<any>) {
if (e.key === "Enter") {
send({ message, user });
}
}
function buttonClick(e: React.MouseEvent<any>) {
send({ message, user });
}
return (
<div className="chat-interface">
<h3>User: {user} </h3>
<input
value={message}
onChange={onChange}
onKeyPress={keyPress}
className="chat-input"
placeholder="Type a message..."
/>
<button onClick={buttonClick}>Send</button>
</div>
);
};
const mapDispatchToProps = (dispatch: Dispatch) => ({
send: ({ message, user }: { message: string; user: string }) =>
message &&
dispatch(
sendMessage({
message,
user,
timestamp: new Date().getTime(),
})
),
updateMessage: (message: string) => dispatch(updateMessage(message)),
});
const mapStateToProps = (state: RootState, ownProps: OwnProps) => ({
user: state.system.userName,
message: state.chat.messageInput,
});
export default connect<StateProps, DispatchProps, OwnProps, RootState>(
mapStateToProps,
mapDispatchToProps
)(ChatInterface);
I decided to keep the UI logic (mapping events to data) within the component. I believe this gives a fairer comparison, as it is not related to state.
With regards to types: this works great!
- Automatic type inference with these lines of boilerplate (in each connected component):
// ../ChatInput.tsx
interface OwnProps {}
type DispatchProps = ReturnType<typeof mapDispatchToProps>;
type StateProps = ReturnType<typeof mapStateToProps>;
type Props = DispatchProps & StateProps & OwnProps;
- Automatic store type inference with this little snippet
// ../store/index.ts
export type RootState = ReturnType<typeof rootReducer>;
TypeScript tells me if my store value has the wrong type when added to JSX, and also when passing the wrong input type into action payloads. It’s good!
It seems I have to explicitly type my action return types, which seems a bit redundant, but it’s nevertheless a part that is unrelated to hooks or not, so I’ll ignore that.
Part III: Converting to hooks
ChatHistory: replace with hooks
Diff: 1310a50
ChatHistory only used State. I feel the readability of the code is better, and it’s also shorter, going from 29 to 21 lines.
Sidenote in case you come across it too: I believed for a second that we rendered twice instead of once when doing the swap, but that’s a debug-feature.
ChatInput: replace with hooks
Diff: 988ee06
ChatInput went from 70 to 57 lines, with a total codediff of -13 lines (being the only changed file). I still decided to keep the UI-related logic outside of hooks, so the difference isn’t as large as it could be.
Again, I think the diff makes the component read better as there is less boilerplate code, ala
interface OwnProps {}
type DispatchProps = ReturnType<typeof mapDispatchToProps>;
type StateProps = ReturnType<typeof mapStateToProps>;
type Props = DispatchProps & StateProps & OwnProps;
Even without this typing-related code, the inference is intact.
ChatInput: replace hooks with custom hooks
Diff: 1c5d82f
ChatInput goes from 57 to 34 lines, but since we’re adding two new hooks files, we end up with a +14 code line change. With custom hooks, we can rename things as we please, and all we end up with (relating to redux) is:
const { inputValue, setInputValue, submit } = useChatInput();
const { userName } = useAuth();
It does require us to add (and maintain) extra “hooks files”, but I think it reads very easily.
The separation of concerns are clear, with clean ability to reuse logic across components. Though this commit is some extra lines of code, it could become fewer if the hooks are reused; even just once.
Summary
The overall change from MapXToProps to using built-in hooks can be seen in the diff c22c184…988ee06
The change from MapToProps to using custom hooks can be seen in the diff 1310a50…1c5d82f
-
Type checking was preserved throughout the changes.
-
Code size decreased when changing to built-in hooks.
-
Code size was equal when changing to custom hooks.
-
Component with hooks will rerender when parent rerenders, unlike with MapXToProps. However, this can easily be fixed with
React.useMemo
wrapping the component.
Overall, I do not see good reasons to keep using MapXToProps. Hooks seem more consise and readable.